Glide Like a Pro: How I Made Smooth Scrolling Magic in Next.js with Lenis and GSAP
Alright, picture this: it’s 2 AM, my second cup of coffee has gone cold, and I’m sitting there, trying to figure out why my Next.js app scrolls like a PowerPoint presentation from 2005. (You know the ones, where every transition looks like it’s running on a dial-up connection.)
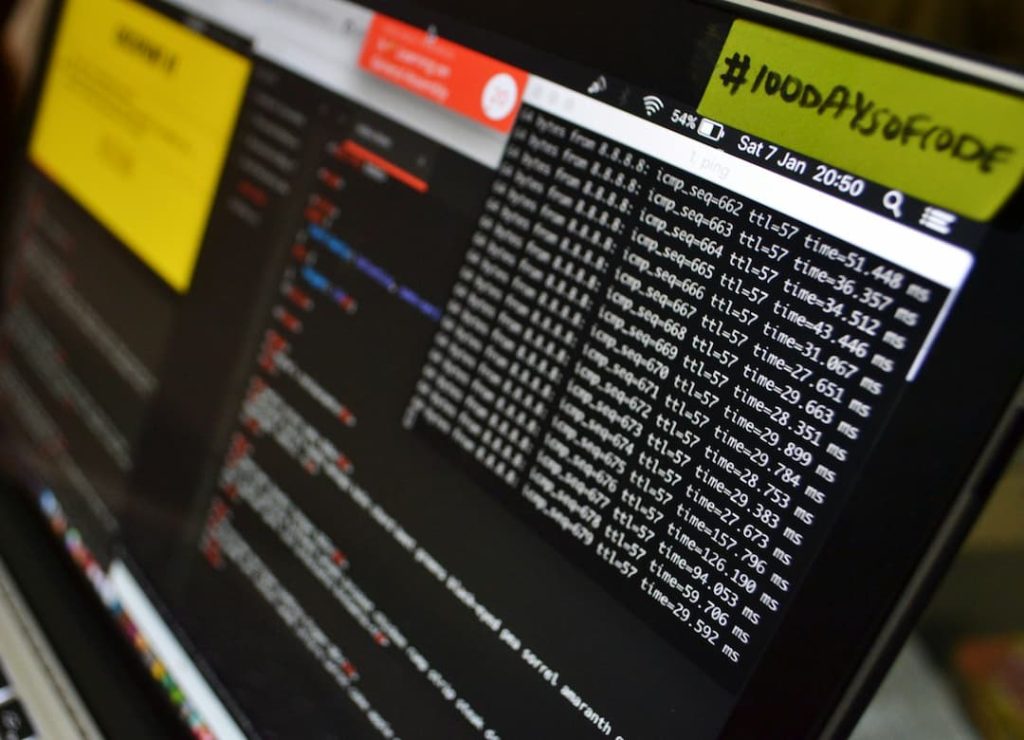
If you’re into web development, you get how satisfying it is when your app scrolls so smoothly it feels like butter melting on a hot pan. Well, that’s exactly what I wanted. I didn’t want a scroll; I wanted an experience. And guess what? I found the magic combo: Lenis and GSAP.
Let me break it down for you, step by step, like we’re hanging out and coding together. (Trust me, by the end of this, you’ll be adding smooth scrolling to everything—even your shopping cart app.)
- See also: How I Nailed Fetching User Location in React.js (Without Losing My Mind 😅)
The Backstory: Why Smooth Scrolling?
First, let’s talk about why smooth scrolling matters. Sure, it’s one of those “small touches,” but in the world of UX, small touches are what separate meh from chef’s kiss.
When users scroll, you want them to feel like they’re gliding through your content effortlessly, not like they’re navigating a laggy mess. And if you’re building anything remotely creative—portfolios, blogs, or SaaS dashboards—smooth scrolling is like adding that perfect Instagram filter.
So, after a lot of Googling (and cursing at janky scroll attempts), I stumbled upon Lenis, a smooth scrolling library that’s lightweight, performant, and surprisingly easy to work with. Add GSAP into the mix for animations, and you’re golden.
Step 1: Setting Up Your Next.js Project
First things first, you need a Next.js app. If you don’t already have one, fire up your terminal and run:
npx create-next-app@latest smooth-scroll-demo
cd smooth-scroll-demo
npm install
Now you’ve got your boilerplate. Open up your favorite code editor (shoutout to VS Code gang 👋), and let’s get cracking.
Step 2: Installing Lenis and GSAP
This is where the magic begins. Install both libraries:
npm install @studio-freight/lenis gsap
For those new to Lenis, think of it as a scroll manager that smooths out the user experience without needing 10,000 lines of code. And GSAP? It’s the animation wizard that’ll make your scroll transitions pop.
Step 3: Setting Up Lenis
Here’s the cool thing about Lenis: it’s ridiculously easy to integrate. Create a new file, lenis.js
, inside a folder like /lib
or /utils
(up to you).
import Lenis from '@studio-freight/lenis';
export const initLenis = () => {
const lenis = new Lenis({
duration: 1.2,
easing: (t) => Math.min(1, 1.001 - Math.pow(2, -10 * t)), // Custom easing curve
smooth: true,
});
function raf(time) {
lenis.raf(time);
requestAnimationFrame(raf);
}
requestAnimationFrame(raf);
return lenis;
};
Now, head over to your _app.js
file and initialize Lenis:
import { useEffect } from 'react';
import { initLenis } from '../utils/lenis';
function MyApp({ Component, pageProps }) {
useEffect(() => {
const lenis = initLenis();
return () => lenis.destroy(); // Clean up on unmount
}, []);
return <Component {...pageProps} />;
}
export default MyApp;
At this point, your app should already feel smoother. Take a moment to scroll and soak it in. (No, seriously—take a break. You’ve earned it.)
Step 4: Adding GSAP for Animations
Here’s where the real fun begins. GSAP makes animations feel so seamless it’s like sprinkling fairy dust on your project. Install the ScrollTrigger plugin:
npm install gsap
In index.js
(or wherever your main content lives), let’s set up a basic animation.
import { useEffect } from 'react';
import { gsap } from 'gsap';
import { ScrollTrigger } from 'gsap/ScrollTrigger';
gsap.registerPlugin(ScrollTrigger);
export default function Home() {
useEffect(() => {
const sections = document.querySelectorAll('.section');
gsap.fromTo(
sections,
{ opacity: 0, y: 50 },
{
opacity: 1,
y: 0,
duration: 1,
ease: 'power2.out',
stagger: 0.2,
scrollTrigger: {
trigger: sections,
start: 'top 80%',
end: 'bottom 20%',
scrub: true,
},
}
);
}, []);
return (
<div>
<div className="section" style={{ height: '100vh', background: '#f3f4f6' }}>
<h1>Welcome to Smooth Scrolling</h1>
</div>
<div className="section" style={{ height: '100vh', background: '#e5e7eb' }}>
<h2>Scroll down to see the magic ✨</h2>
</div>
<div className="section" style={{ height: '100vh', background: '#d1d5db' }}>
<h2>That’s all, folks!</h2>
</div>
</div>
);
}
Step 5: Fine-Tuning and Debugging
Here’s the thing—smooth scrolling can be a bit extra. You’ll need to tweak values like duration
, scrub
, and easing curves to get it just right.
For me, the sweet spot was a 1.2
duration and a custom easing function (because why not?). Also, don’t forget to test on different devices—what looks amazing on desktop might feel sluggish on mobile.
Pro tip: If your animations feel “off,” check your CSS. Sometimes, overflow or z-index issues can mess with the scroll experience.
Step 6: Celebrate! 🎉
That’s it—you’ve officially mastered smooth scrolling in Next.js using Lenis and GSAP. Pat yourself on the back, blast your favorite playlist, and marvel at how silky smooth your app feels now.
Final Thoughts
If you’ve made it this far, you’re basically a scroll wizard. But don’t stop here—Lenis and GSAP are just the beginning. Experiment with parallax effects, interactive scroll-triggered animations, and more.
And hey, if you run into any issues, drop a comment below. I’ll probably be up at 2 AM again, coffee in hand, ready to help. 😉
Until next time, keep scrolling smooth, my friends. ✌️
Discover more from Rabbit Rank
Subscribe to get the latest posts sent to your email.