How to Create an Awesome Pricing Section with Tailwind CSS in React
Let me set the scene for you: it’s 10 p.m., and I’m halfway through a tub of Ben & Jerry’s Cherry Garcia. My client—a startup with a brand voice that screams “We’re quirky but professional!”—just requested a pricing section for their app. Simple, right? Except they wanted it to be responsive, visually appealing, and ready yesterday. Classic.
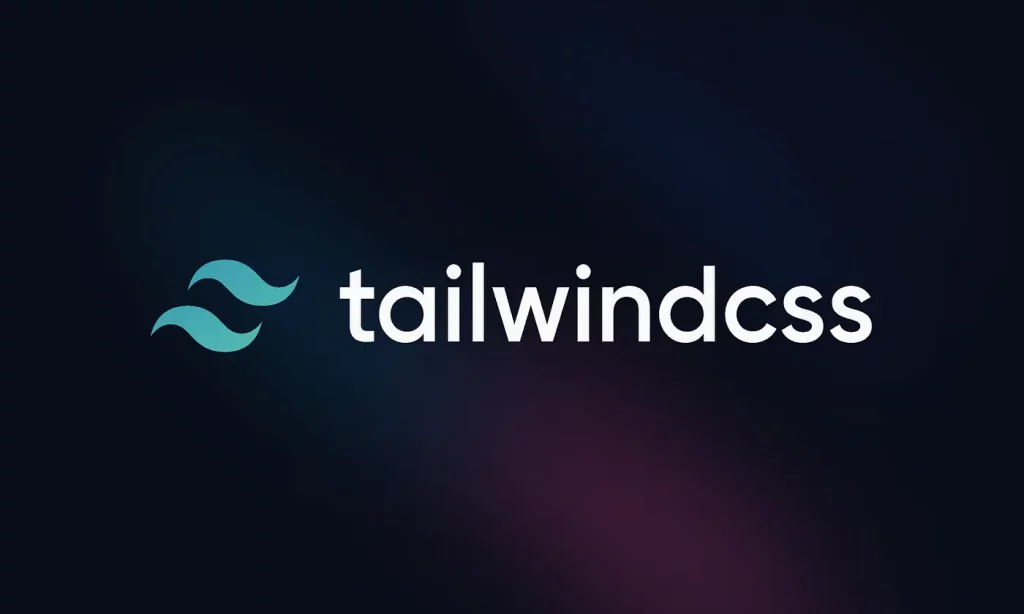
If you’ve ever been in the “I’ll code my way out of this” club, welcome. In today’s post, I’m going to walk you through creating a sleek pricing section using Tailwind CSS and React. Bonus: we’ll keep the code as clean as your mom’s kitchen counters (if she’s anything like mine).
Step 1: Understand What We’re Building
We’ll create a pricing component with two plans: Hobby and Enterprise. The Hobby tier is for newcomers, while the Enterprise tier has all the bells and whistles. Each tier will include features, a price, and a CTA button. Oh, and we’ll add some tasteful gradients because “modern design”.
Here’s the design breakdown:
- Hobby Plan: Simple and approachable.
- Enterprise Plan: Sleek, feature-packed, and gets extra love with shadows and colors.
Step 2: Set Up Your Project
You’ll need a React project with Tailwind CSS installed. If you’re starting from scratch, run this in your terminal:
npx create-react-app pricing-component
cd pricing-component
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init
Update your tailwind.config.js
and include Tailwind’s directives in your src/index.css
:
@tailwind base;
@tailwind components;
@tailwind utilities;
Once your setup is good to go, fire up the dev server with npm start
. Time to code!
Step 3: The Code (Finally!)
Here’s the component we’ll build. Copy this into a new file—PricingSection.js
:
import { CheckIcon } from '@heroicons/react/20/solid';
const tiers = [
{
name: 'Hobby',
id: 'tier-hobby',
href: '#',
priceMonthly: '$29',
description: "The perfect plan if you're just getting started with our product.",
features: ['25 products', 'Up to 10,000 subscribers', 'Advanced analytics', '24-hour support response time'],
featured: false,
},
{
name: 'Enterprise',
id: 'tier-enterprise',
href: '#',
priceMonthly: '$99',
description: 'Dedicated support and infrastructure for your company.',
features: [
'Unlimited products',
'Unlimited subscribers',
'Advanced analytics',
'Dedicated support representative',
'Marketing automations',
'Custom integrations',
],
featured: true,
},
];
function classNames(...classes) {
return classes.filter(Boolean).join(' ');
}
export default function PricingSection() {
return (
<div className="relative isolate bg-white px-6 py-24 sm:py-32 lg:px-8">
<div className="mx-auto max-w-4xl text-center">
<h2 className="text-base font-semibold text-indigo-600">Pricing</h2>
<p className="mt-2 text-5xl font-semibold tracking-tight text-gray-900 sm:text-6xl">
Choose the right plan for you
</p>
</div>
<p className="mx-auto mt-6 max-w-2xl text-center text-lg text-gray-600">
Choose an affordable plan that’s packed with the best features for engaging your audience, creating customer loyalty, and driving sales.
</p>
<div className="mx-auto mt-16 grid max-w-lg grid-cols-1 gap-y-6 lg:max-w-4xl lg:grid-cols-2">
{tiers.map((tier) => (
<div
key={tier.id}
className={classNames(
tier.featured ? 'relative bg-gray-900 shadow-2xl' : 'bg-white/60',
'rounded-3xl p-8 ring-1 ring-gray-900/10'
)}
>
<h3 className={classNames(tier.featured ? 'text-indigo-400' : 'text-indigo-600', 'text-base font-semibold')}>
{tier.name}
</h3>
<p className="mt-4 text-5xl font-semibold text-gray-900">{tier.priceMonthly}</p>
<p className="mt-6 text-gray-600">{tier.description}</p>
<ul className="mt-8 space-y-3 text-sm text-gray-600">
{tier.features.map((feature) => (
<li key={feature} className="flex gap-x-3">
<CheckIcon className="h-6 w-5 text-indigo-600" />
{feature}
</li>
))}
</ul>
<a
href={tier.href}
className="mt-8 block rounded-md bg-indigo-500 px-3.5 py-2.5 text-center text-sm font-semibold text-white hover:bg-indigo-400"
>
Get started today
</a>
</div>
))}
</div>
</div>
);
}
Step 4: Customize and Style
Tailwind gives you a strong base, but feel free to tweak the colors, typography, or spacing to match your brand. Want a different gradient? Swap it out in the bg-gradient-to-tr
class. Need rounded corners for days? Bump up the rounded-3xl
to rounded-full
. Flex your creativity.
Step 5: Test and Deploy
Don’t forget to test on multiple devices. Tailwind’s responsive utilities (e.g., sm:
, lg:
) are a lifesaver. Once you’re satisfied, deploy your app with your favorite hosting service—Vercel, Netlify, you name it.
Wrap-Up
And there you have it—a responsive, visually pleasing pricing section built with Tailwind CSS and React. If your client’s jaw doesn’t drop when they see it, at least you know it’s awesome. 😉
Got questions or want to share your version of this component? Drop me a comment below or hit me up on Twitter. Let’s geek out about code together!